Learn Python
This course is designed to introduce students to the fundamentals of programming using Python.
Students will learn core programming concepts, Python syntax, and how to write efficient and effective code.
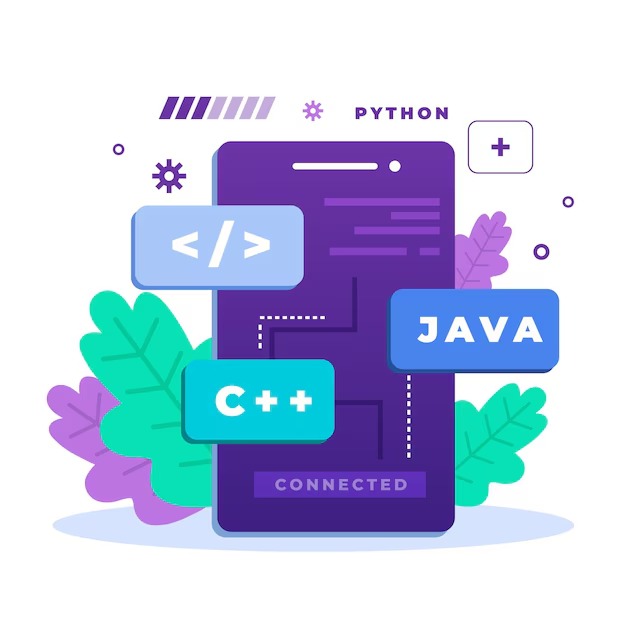
Python Programming Course Syllabus
Course Description
This course is designed to introduce students to the fundamentals of programming using Python. Students will learn core programming concepts, Python syntax, and how to write efficient and effective code.
Course Objectives
By the end of this course, students will be able to:
- Understand basic programming concepts
- Write and execute Python programs
- Use Python data types and control structures
- Define and use functions
- Work with files and handle exceptions
- Understand object-oriented programming concepts in Python
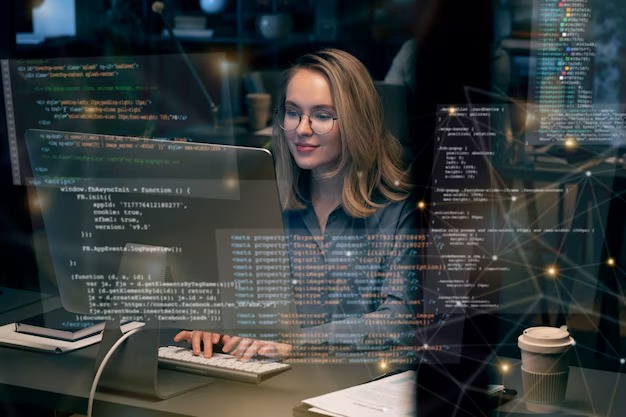
Course Outline
Week 1: Introduction to Python
- What is Python?
- Setting up the Python environment
- Writing your first Python program
- Basic syntax and data types
Week 2: Control Structures
- Conditional statements (if, elif, else)
- Loops (for, while)
- List comprehensions
Week 3: Data Structures
- Lists and tuples
- Dictionaries and sets
- Working with strings
Week 4: Functions
- Defining and calling functions
- Parameters and return values
- Lambda functions
- Modules and packages
Week 5: File Handling and Exceptions
- Reading and writing files
- Working with CSV and JSON
- Exception handling (try, except, finally)
Week 6: Object-Oriented Programming
- Classes and objects
- Inheritance and polymorphism
- Encapsulation and abstraction
Week 7: Advanced Topics
- List comprehensions and generators
- Decorators
- Context managers
Week 8: Final Project
- Students will work on a final project applying the concepts learned throughout the course
Assessment
- Weekly coding assignments (40%)
- Midterm exam (20%)
- Final project (30%)
- Class participation (10%)
Required Materials
- Python 3.x installed on your computer
- A text editor or Integrated Development Environment (IDE) of your choice
- Course textbook (TBD)