Learn JavaScript Programming
This course is designed to provide a comprehensive introduction to the JavaScript programming language.
Students will learn the fundamentals of JavaScript, including its syntax, data types, control structures, and object-oriented programming concepts.

Learn SQL Programming Course Syllabus
Course Description
This course is designed to provide a comprehensive introduction to the JavaScript programming language. Students will learn the fundamentals of JavaScript, including its syntax, data types, control structures, and object-oriented programming concepts. The course will also cover the use of JavaScript in both client-side and server-side (Node.js) web development.
Course Objectives
By the end of this course, students will be able to:
- Understand the role and history of JavaScript in web development
- Write, test, and debug JavaScript code in both client-side and server-side environments
- Work with JavaScript data types, variables, operators, and expressions
- Implement control structures (conditional statements, loops) in JavaScript
- Define and use functions, including arrow functions and closures
- Understand and apply object-oriented programming concepts in JavaScript
- Manipulate the Document Object Model (DOM) and handle events
- Develop basic web applications using JavaScript and HTML/CSS
- Explore modern JavaScript features (ES6+) and their usage
- Build simple server-side applications using Node.js and Express
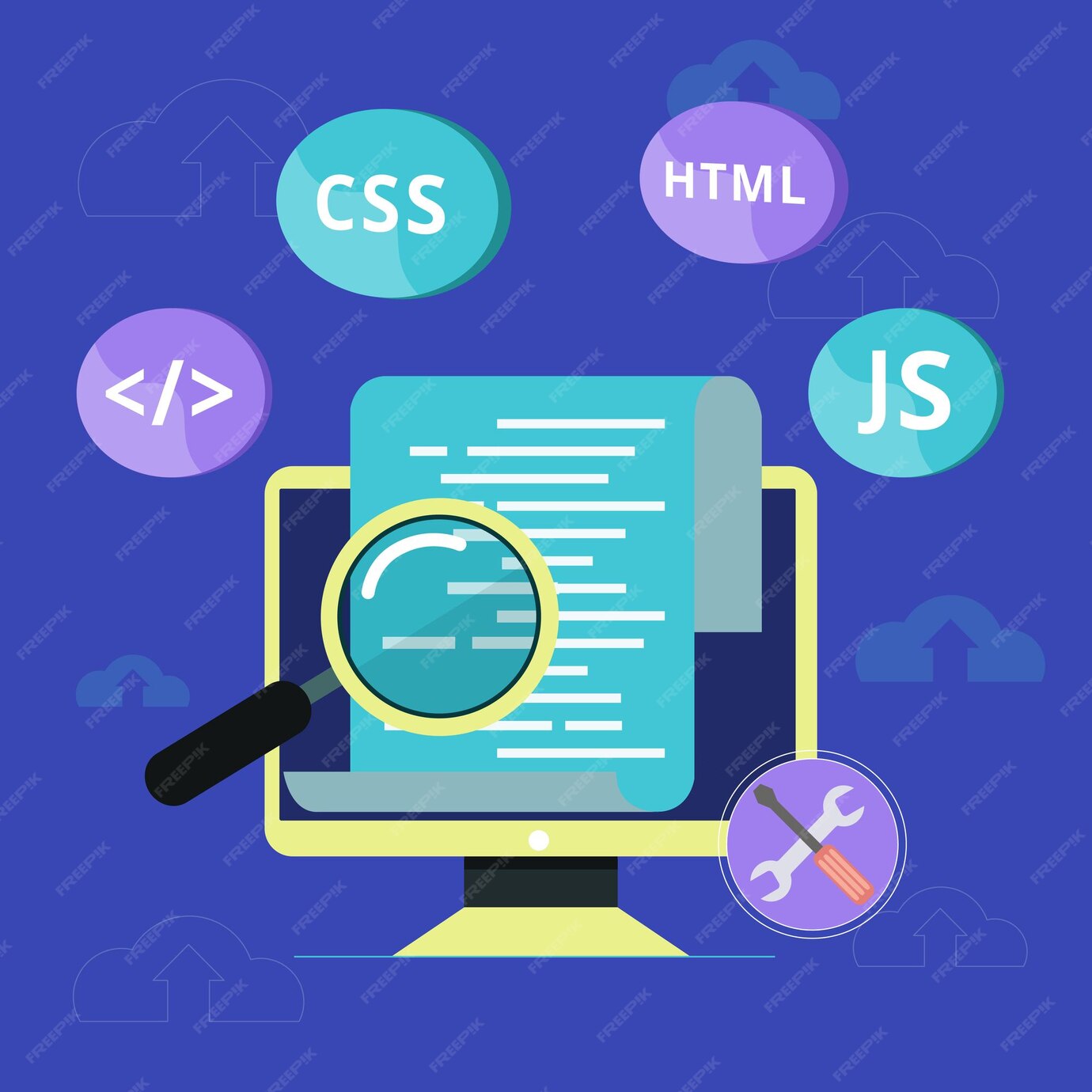
Course Outline
Week 1: Introduction to JavaScript
- What is JavaScript? History and evolution
- Setting up a development environment
- Writing your first JavaScript program
- JavaScript in the browser and on the server (Node.js)
Week 2: JavaScript Syntax and Data Types
- JavaScript syntax and coding conventions
- Primitive data types (number, string, boolean, etc.)
- Variables, constants, and type coercion
- Operators and expressions
Week 3: Control Structures and Functions
- Conditional statements (if-else, switch)
- Looping constructs (for, while, do-while)
- Functions, function expressions, and arrow functions
- Scope and closure
Week 4: Objects and Arrays
- Creating and manipulating objects
- Object properties and methods
- Array creation, indexing, and methods
- Object-oriented programming in JavaScript
Week 5: DOM Manipulation and Events
- Document Object Model (DOM) structure
- Selecting and modifying DOM elements
- Handling user events (click, mouseover, etc.)
- Event propagation and delegation
Week 6: Asynchronous JavaScript
- Callbacks and the event loop
- Promises and async/await
- Fetch API and making HTTP requests
- Timers (setTimeout, setInterval)
Week 7: Modern JavaScript and Node.js
- ES6+ features (let, const, template literals, etc.)
- Modules and import/export
- Introduction to Node.js and the server-side
- Building a simple web server with Express
Week 8: Final Project
- Students will build a web application, combining client-side and server-side JavaScript concepts learned throughout the course
Assessment
- Weekly coding assignments (40%)
- Midterm project (20%)
- Final project (30%)
- Class participation and code reviews (10%)