Learn Advanced Java
This course is designed to provide a deep dive into advanced Java programming concepts and techniques.
Building upon the foundations of core Java, students will explore more complex language features, design patterns, and enterprise-level application development.

Advanced Java Programming Course Syllabus
Course Description
This course is designed to provide a deep dive into advanced Java programming concepts and techniques. Building upon the foundations of core Java, students will explore more complex language features, design patterns, and enterprise-level application development.
Course Objectives
By the end of this course, students will be able to:
- Understand and utilize Java 8+ language features (lambda expressions, streams, etc.)
- Implement various design patterns in Java (creational, structural, and behavioral)
- Work with concurrency and multithreading in Java
- Apply Java I/O, NIO, and networking APIs
- Develop a basic understanding of Java Enterprise Edition (Java EE)
- Utilize Java’s reflective capabilities and annotation processing
- Explore Java testing frameworks and practices
- Understand Java performance optimization and profiling
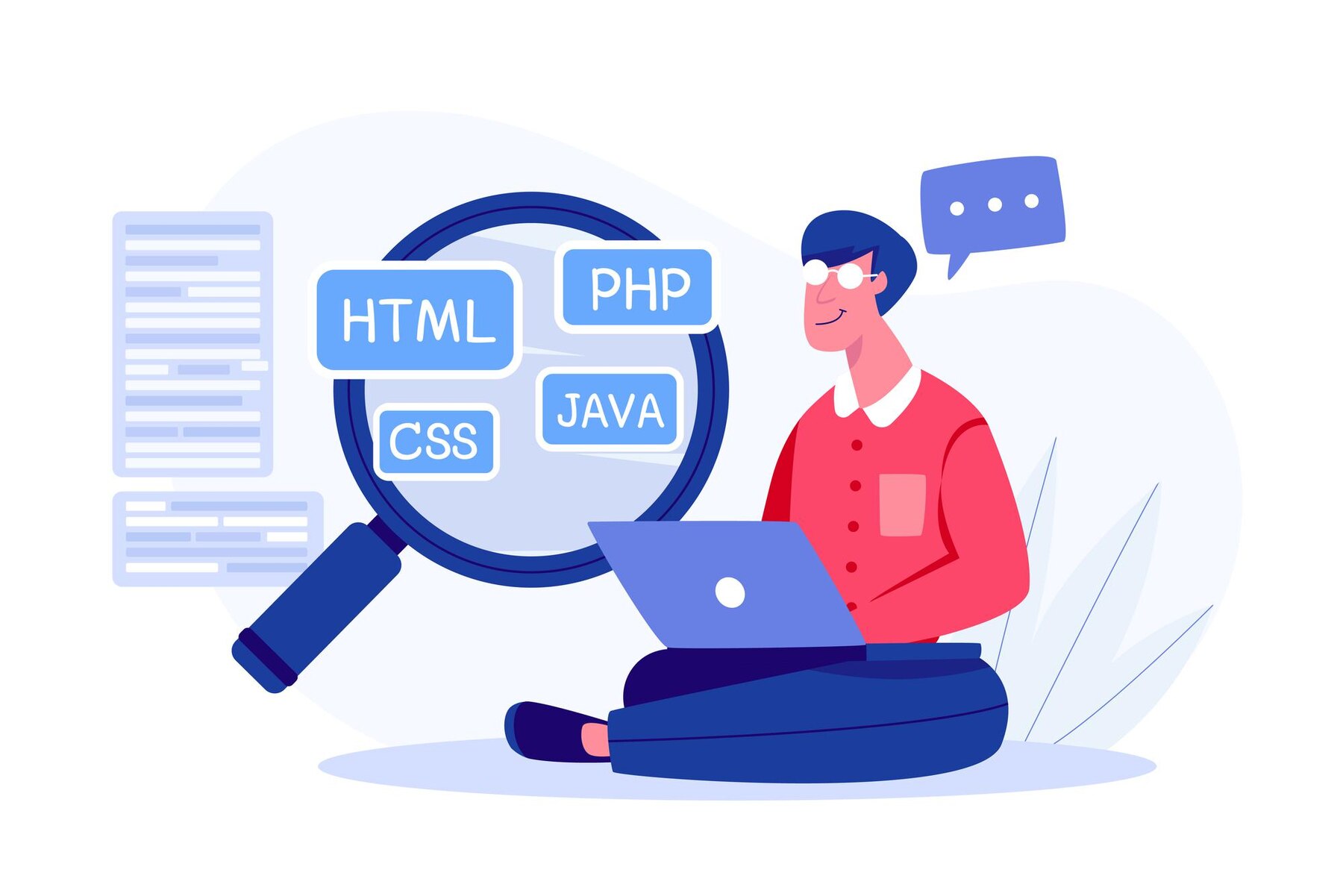
Course Outline
Week 1: Java 8+ Language Features
- Lambda expressions and functional interfaces
- Method references
- Stream API and common stream operations
- Date and Time API
Week 2: Design Patterns in Java
- Creational patterns (Singleton, Factory, Builder)
- Structural patterns (Adapter, Decorator, Proxy)
- Behavioral patterns (Observer, Strategy, Command)
- Applying design patterns in Java programs
Week 3: Concurrency and Multithreading
- Thread creation and lifecycle management
- Synchronization and thread safety
- Concurrent collections and thread-safe data structures
- Executors, Futures, and Completable Futures
Week 4: Java I/O and NIO
- Byte streams and character streams
- File I/O and working with paths
- NIO.2 and its enhancements (async I/O, etc.)
- Networking with Java Sockets and URL handling
Week 5: Java Enterprise Edition (Java EE)
- Introduction to Java EE and its core components
- Servlets and web application development
- Enterprise JavaBeans (EJB) and dependency injection
- Java Persistence API (JPA) and database integration
Week 6: Reflection and Annotation Processing
- Java Reflection API and its applications
- Custom annotation creation and processing
- Generating code at compile-time with annotation processors
Week 7: Testing and Profiling
- JUnit and unit testing best practices
- Mocking and test-driven development
- Performance optimization and profiling tools
- Monitoring and troubleshooting Java applications
Week 8: Final Project
- Students will work on a comprehensive project that integrates the advanced Java concepts learned throughout the course
Assessment
- Weekly coding assignments (30%)
- Midterm project (20%)
- Final project (40%)
- Class participation and presentations (10%)