Learn Core Java
This course is designed to provide a comprehensive introduction to the Java programming language.
Students will learn the fundamentals of Java, including its syntax, data structures, control flow, and object-oriented programming concepts.
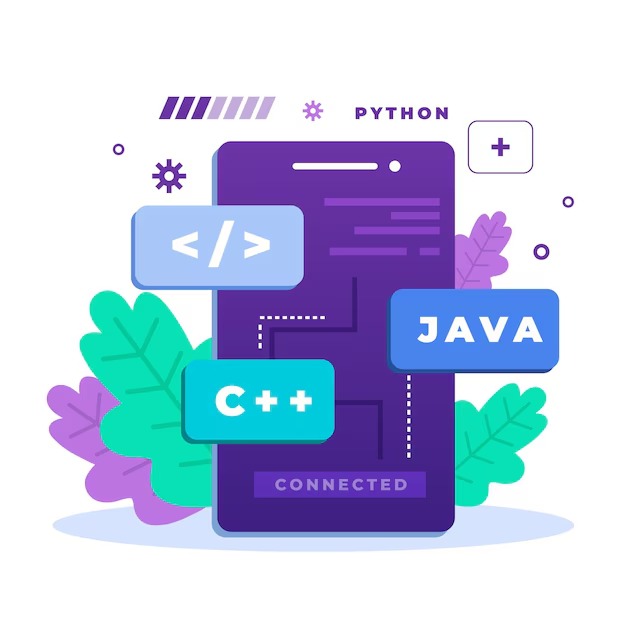
Core Java Programming Course Syllabus
Course Description
This course is designed to provide a comprehensive introduction to the Java programming language. Students will learn the fundamentals of Java, including its syntax, data structures, control flow, and object-oriented programming concepts. The course will also cover essential Java APIs and tools for application development.

Course Objectives
By the end of this course, students will be able to:
- Understand the history and evolution of the Java programming language
- Write, compile, and execute Java programs
- Work with primitive data types, operators, and expressions
- Use control structures like conditional statements and loops
- Implement and utilize arrays, strings, and other data structures
- Define and use methods, classes, and objects
- Understand inheritance, polymorphism, and encapsulation
- Work with exceptions, file I/O, and Java collections
- Develop a basic understanding of Java standard library and APIs
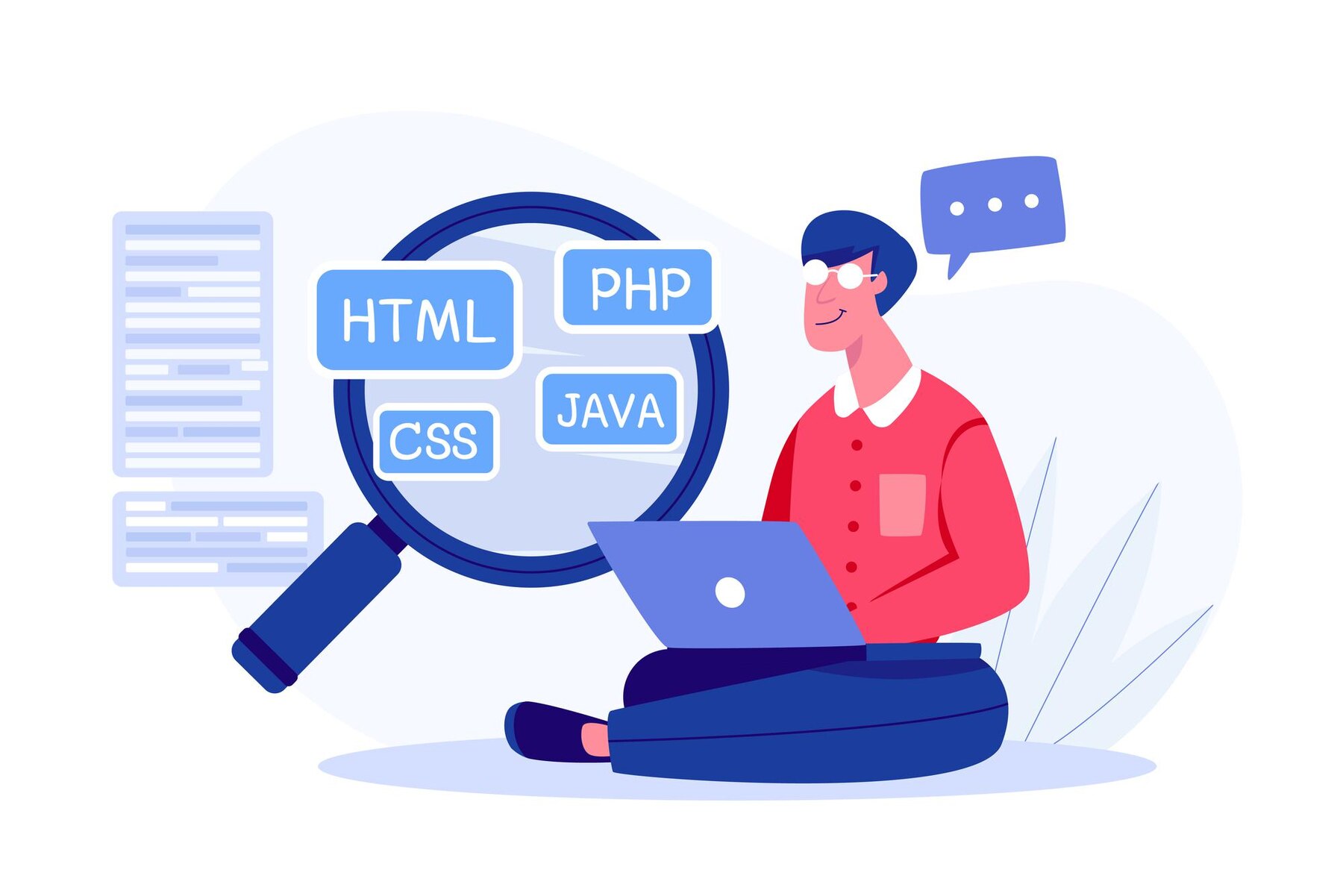
Course Outline
Week 1: Introduction to Java
- What is Java?
- History and evolution of Java
- Java development environment (JDK, IDE)
- Writing and executing your first Java program
Week 2: Java Syntax and Data Types
- Java syntax and coding conventions
- Primitive data types (int, double, boolean, etc.)
- Variables, literals, and type casting
- Operators and expressions
Week 3: Control Structures
- Conditional statements (if-else, switch)
- Looping constructs (for, while, do-while)
- Break, continue, and return statements
Week 4: Arrays and Strings
- Declaring and initializing arrays
- Array operations (sorting, searching, etc.)
- Working with strings and string manipulation
Week 5: Methods and Classes
- Defining and calling methods
- Method overloading and varargs
- Classes, objects, and constructors
- Access modifiers and encapsulation
Week 6: Inheritance and Polymorphism
- Inheritance and class hierarchy
- Method overriding and dynamic method dispatch
- Abstract classes and interfaces
Week 7: Exception Handling and File I/O
- Exception types and the try-catch-finally block
- Throwing and handling exceptions
- Reading and writing files
- Working with Java I/O classes
Week 8: Java Collections and APIs
- Overview of the Java Collections Framework
- Lists, sets, maps, and their implementations
- Exploring common Java standard library APIs
- Building a final project utilizing the concepts learned
Assessment
- Weekly coding assignments (40%)
- Midterm exam (20%)
- Final project (30%)
- Class participation and code reviews (10%)